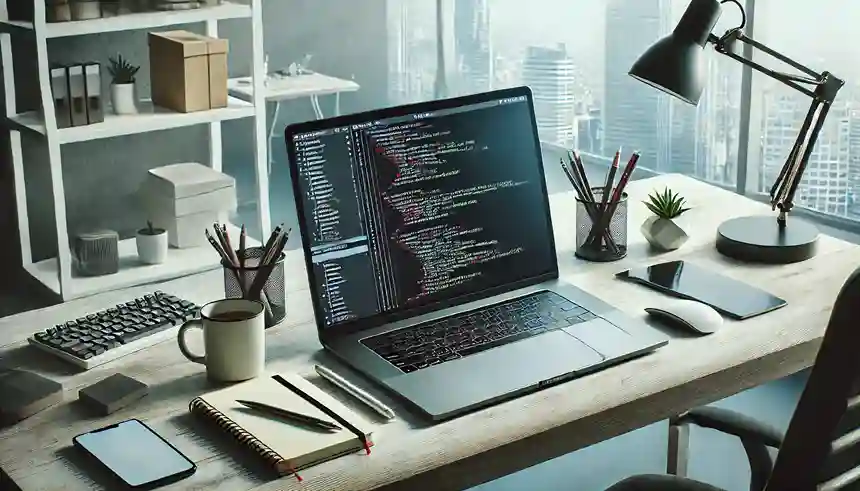
Android DataStore 개요 및 사용 방법
1. DataStore란?
DataStore는 안드로이드에서 데이터를 저장하는 방법 중 하나로, SharedPreferencses의 대안으로 설계되었습니다. DataStore는 더욱 안전하고 비동기적으로 작동하며, 프로토콜 버퍼(Protobuf) 또는 JSON을 사용해 데이터를 저장합니다. DataStore는 Preferences DataStore 와 Proto DataStore 두 가지 유형으로 제공됩니다.
- Preferences DataStore: SharedPreferences와 유사한 방식으로 간단한 키-값 데이터를 저장합니다.
- Proto DataStore: 구조화된 데이터를 저장할 때 사용되며, Protobuf를 통해 데이터를 직렬화합니다.
2. DataStore 설치
DataStore를 사용하기 위해 build.gradle 파일에 의존성을 추가하고, Sync Now를 클릭하여 의존성을 다운로드합니다.
dependencies {
implementation "androidx.datastore:datastore-preferences:1.0.0"
implementation "androidx.datastore:datastore:1.0.0"
}
3. Preferences DataStore 사용 예시
Preferences DataStore는 간단한 키-값 저장소로, SharedPreferences와 비슷하지만 비동기적으로 동작하여 성능이 향상됩니다. 예시로, 사용자 설정 값을 저장하는 방법을 살펴보겠습니다.
1) DataStore 객체 생성
DataStore는 Context를 통해 생성합니다. Preferences 클래스는 String, Int, Boolean, Float, Long 타입의 데이터를 저장할 수 있습니다.
val Context.dataStore: DataStore<Preferences> by preferencesDataStore(name = "settings")
2) 데이터 저장
데이터를 저장할 때는 edit 메서드를 사용하여 데이터를 변경합니다. edit은 비동기적으로 실행되므로, 코루틴을 통해 호출해야 합니다.
import androidx.datastore.preferences.edit
import androidx.datastore.preferences.preferencesKey
val EXAMPLE_KEY = preferencesKey<String>("example_key")
suspend fun saveData(context: Context, value: String) {
context.dataStore.edit { preferences ->
preferences[EXAMPLE_KEY] = value
}
}
3) 데이터 읽기
데이터를 읽을 때는 data 프로퍼티를 사용하여 값을 가져옵니다. 마찬가지로, Flow로 반환되므로 collect를 통해 값을 비동기적으로 받을 수 있습니다.
import kotlinx.coroutines.flow.first
suspend fun readData(context: Context): String? {
val preferences = context.dataStore.data.first()
return preferences[EXAMPLE_KEY]
}
4. Proto DataStore 사용 예시
Proto DataStore는 Proto 객체를 사용하여 구조화된 데이터를 저장합니다. Protobuf를 통해 데이터 직렬화 및 역직렬화가 가능하므로, 더 복잡한 데이터를 안전하고 효율적으로 저장할 수 있습니다.
1) Protobuf 정의
먼저 .proto 파일을 정의해야 합니다. proto 파일을 프로젝트에 추가하고, Protofub 플러그인을 설정합니다.
build.gradle 파일에 다음과 같은 의존성을 추가하고, 예시로 User.proto 파일을 만듭니다.
(1) build.gradle
plugins {
id 'com.google.protobuf' version '0.9.1'
}
dependencies {
implementation 'com.google.protobuf:protobuf-javalite:3.19.0'
implementation 'androidx.datastore:datastore-core:1.0.0'
}
(2) User.proto
syntax = "proto3";
option java_package = "com.example.datastore";
message User {
string name = 1;
int32 age = 2;
}
2) Proto 객체 사용
Proto DataStore를 설정하고 데이터를 저장/읽는 방법을 설명합니다.
import androidx.datastore.core.Serializer
import com.example.datastore.User
import com.google.protobuf.InvalidProtocolBufferException
import java.io.InputStream
import java.io.OutputStream
object UserSerializer : Serializer<User> {
override val defaultValue: User = User.getDefaultInstance()
override suspend fun readFrom(input: InputStream): User {
try {
return User.parseFrom(input)
} catch (exception: InvalidProtocolBufferException) {
throw IOException("Error reading data", exception)
}
}
override suspend fun writeTo(t: User, output: OutputStream) {
t.writeTo(output)
}
}
이후 Proto DataStore를 사용하여 데이터를 읽고 저장할 수 있습니다.
val Context.userDataStore: DataStore<User> by dataStore(
fileName = "user.pb",
serializer = UserSerializer
)
suspend fun saveUser(context: Context, user: User) {
context.userDataStore.updateData { user }
}
suspend fun getUser(context: Context): User {
return context.userDataStore.data.first()
}
5. DataStore 활용 방법
DataStore는 비동기적이고 안전한 방식으로 데이터를 관리할 수 있기 때문에 다음과 같은 활용 방법이 있습니다.
- 앱 설정 저장: 사용자 설정, 테마, 알림 설정 등을 저장하여 앱의 상태를 관리합니다.
- 사용자 정보 관리: Proto DataStore를 사용하여 구조화된 데이터를 안전하게 저장하고 관리할 수 있습니다.
- 성능 최적화: DataStore는 SharedPreferences보다 더 효율적이고 비동기적으로 동작하여 성능을 최작화 할 수 있습니다.
6. DataStore 장점
- 비동기적 처리: DataStore는 모든 I/O 작업을 비동기적으로 처리하여 UI 스레드가 차단되지 않도록 합니다.
- 타입 안전: Protobuf와 함께 사용하면 타입 안전을 보장할 수 있습니다.
- 보안: 데이터를 암호화하여 저장할 수 있어 더 안전하게 관리할 수 있습니다.
- 성능: SharedPreferences보다 더 나은 성능을 제공합니다.
DataStore는 안드로이드 앱에서 안전하고 효율적으로 데이터를 저장할 수 있는 좋은 방법입니다. SharedPreferencse를 대체할 수 있으며, Proto DataStore를 사용하여 복잡한 데이터를 구조화하여 저장할 수 있는 기능까지 제공합니다. 이로 인해 데이터 저장 방식의 확장성과 안전성을 크게 향상시킬 수 있습니다.
도움이 되는 다른 게시물
- Android SharedPreferences: https://koreaworldinfo.co.kr/?p=8